C Program to delete consonants from a string
In this program, You will learn how to delete the occurrences of consonants from a string in c.
#include<stdio.h>
#include<string.h>
int main() {
//statement
}
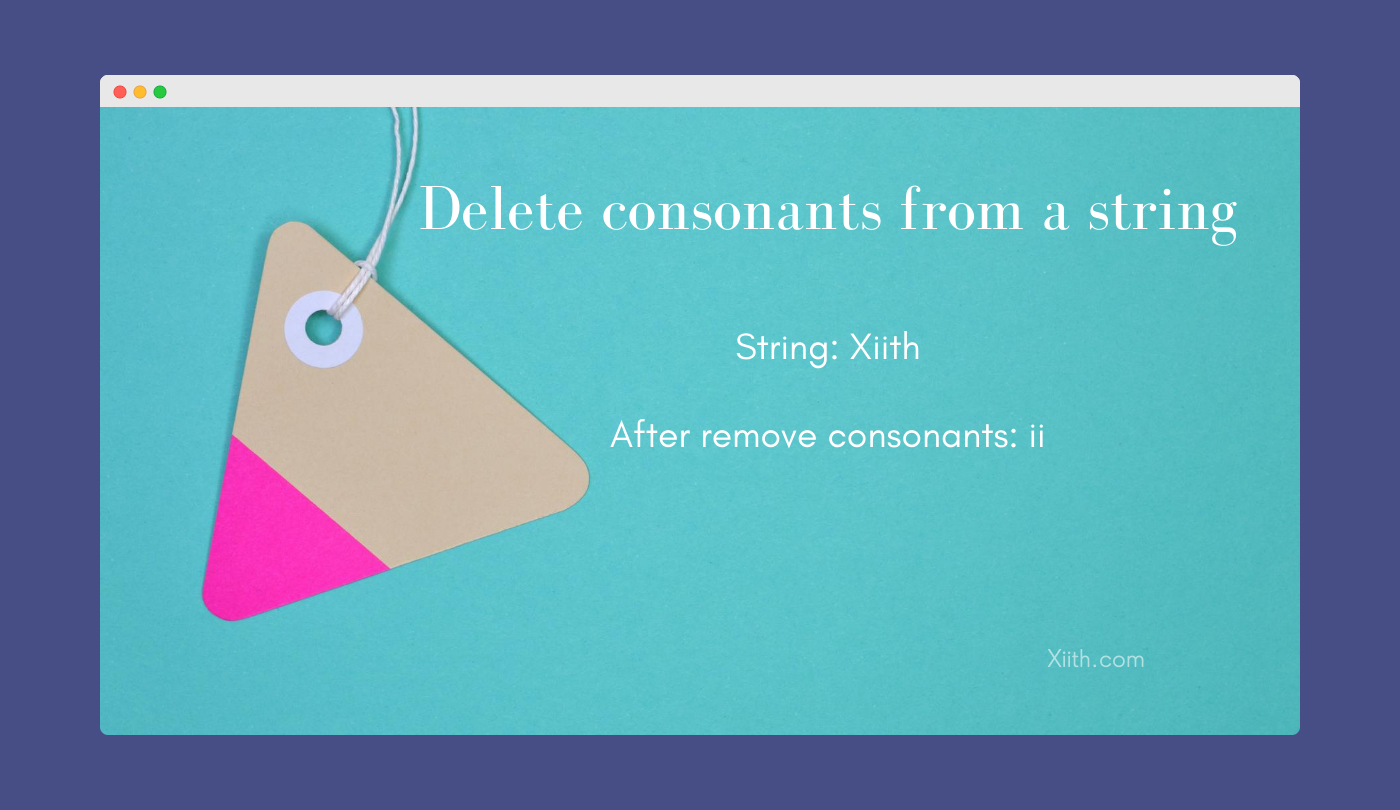
Example: How to delete consonants from a string in c.
#include<stdio.h>
#include<string.h>
int main() {
char str[100], temp[100];
int i = 0, j = 0;
printf("Enter any string:");
scanf("%[^\n]%*c", str);
while (str[i] != '\0') {
if (str[i] == 'a' || str[i] == 'e' ||
str[i] == 'i' || str[i] == 'o' ||
str[i] == 'u' || str[i] == 'A' ||
str[i] == 'E' || str[i] == 'I' ||
str[i] == 'O' || str[i] == 'U' ||
str[i] == 32) {
temp[j++] = str[i];
}
i++;
}
temp[j] = '\0';
strcpy(str, temp);
printf("String without consonant is:%s", str);
return 0;
}
Output:
Enter any string:Xiith
String without consonant is:ii