C Program to find saddle point in a matrix
In this program, You will learn how to find saddle point in a matrix in C.
int a[ 10][10]
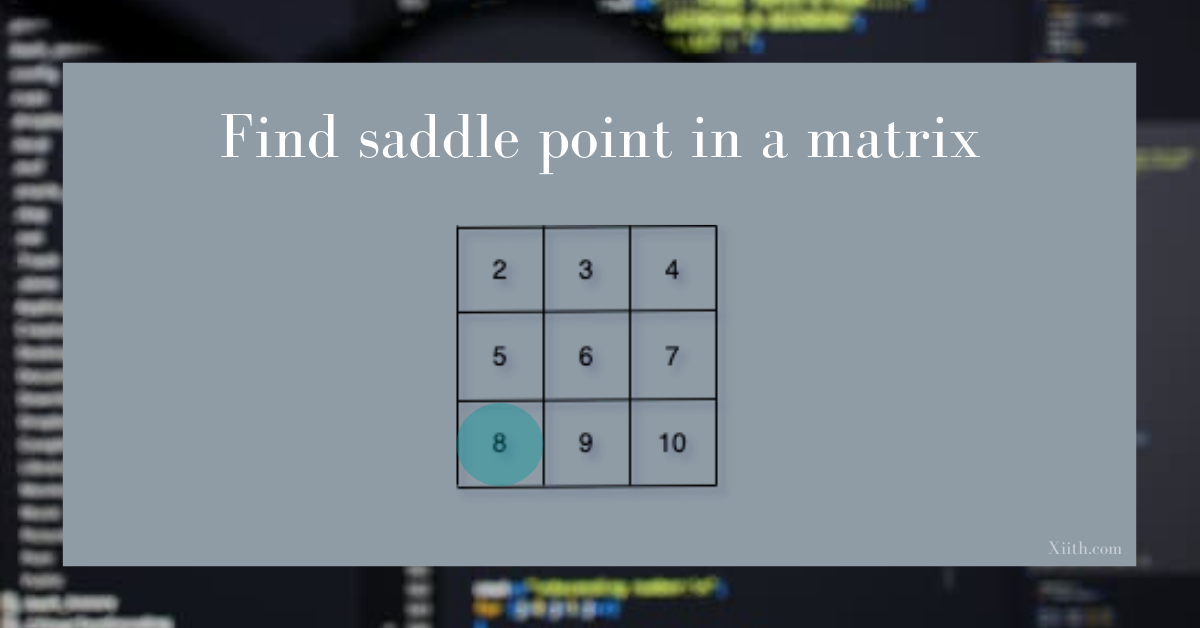
Example: How to find saddle point in a matrix in c.
#include<stdio.h>
int main() {
int a[ 10][10], i, j, num;
int sm, p, large, f = 1;
printf("Enter size of matrix:");
scanf("%d", & num);
printf("Enter 2D array elements:");
for (i = 0; i < num; i++) {
for (j = 0; j < num; j++) {
scanf("%d", & a[i][j]);
}
}
printf("Elements of the 2D array is:");
for (i = 0; i < num; i++) {
printf("\n");
for (j = 0; j < num; j++) {
printf("%d ", a[i][j]);
}
}
/* Logic start here */
for (i = 0; i < num; i++) {
p = 0;
sm = a[i][0];
for (j = 0; j < num; j++) {
if (sm > a[i][j]) {
sm = a[i][j];
p = j;
}
}
large = 0;
for (j = 0; j < num; j++) {
if (large < a[j][p]) {
large = a[j][p];
}
}
if (sm == large) {
printf("\nValue of saddle point:%d", sm);
f = 0;
}
}
if (f > 0)
printf("\nNo saddle point");
return 0;
}
Output:
Enter size of matrix:3
Enter 2D array elements:2 3 4 5 6 7 8 9 10
Elements of the 2D array is:
2 3 4
5 6 7
8 9 10
Value of saddle point:8